Project 2 - Image Operations - (100 points)
Part 1 - Due date: 3/28/2019
Part 2 - Due date: 4/2/2019
Objectives
- Introduction to functions
- Practice with loops
- Introduction to image processing
Introduction
For this project, you will start with the provided program imageoperations.py that takes an input image and creates a copy of the image. Extend the provided started code to add basic image processing functionality to it.
Part 1 (Due 3/28/19)
The operations that you will perform are based on a user's choice shown in the form of a menu. The menu has the following operations:
- Display the menu to the user that contains all the options below. Get input from the user. Note: Remember to perform input validation (5 points)
def flipVertical() (10 points) - flips the image along the horizontal axis, effectively turning it upside down. The new image will be saved to a file called verticalflip.png.
- def findPattern() - (5 points) find the secret pattern in the image. You may remember using a "secret message decoder" toy from the past. Think about a way to get rid of the red "noise" in this image. The new file will be called mystery-solved.png
- def makeGrayscale() (10 points) - converts the image to "black and white" by setting the red, green, and blue value for each pixel to be 30% of the old red value + 59% of the old green value + 11% of the old blue value for that pixel (see: http://en.wikipedia.org/wiki/Grayscale). The new image will be saved to a file grayscale.png.
- def rotate() (10 points) - rotates the image clockwise by 90 degrees. The new image will be saved to a file rotate.png.
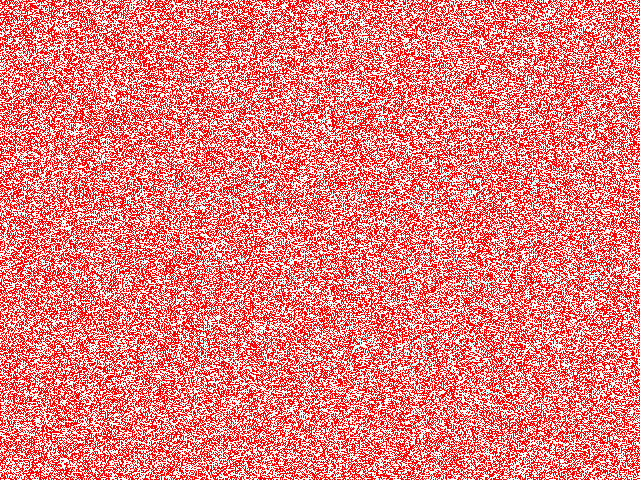
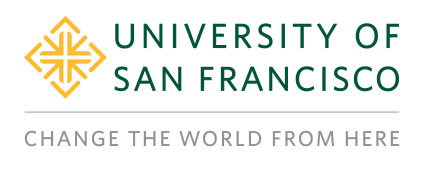
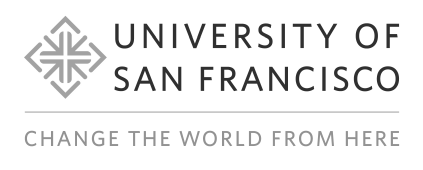
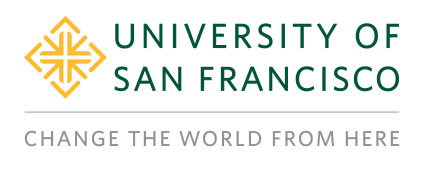
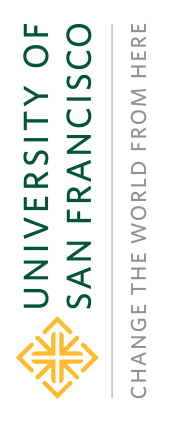
Part 2 (Due 4/2/19)
- def swapCorners() (15 points) - divides the image into four subsquares and moves the upper right to the bottom left and vice versa. The new image will be saved to a file cornerswap.png.
- def blur() - (15 points) blurs the image using the following algorithm: for each pixel, the red value will be set to the average of all red values in a 3x3 square surrounding the pixel, and the same for green and blue. The red value at position (3, 6) would be set to the average of the red values at (2, 5) (3, 5) (4, 5) (2, 6) (3, 6) (4, 6) (2, 7) (3, 7) (4, 7). Make sure to handle the case of a pixel at the edge of the image. The new image will be saved to a file blurred.png. (10 points)
- def sharpenImage() - (15 points) sharpens an existing image using the unsharp mask. For every pixel, calculate the sharpened value using the following formula:
sharpened = original + (original - blurred) * amount. - def edgedetection() - (15 points) extracts the edge from the image using the Sobel edge detection operator. The new file will be saved to a file called sobel.png.
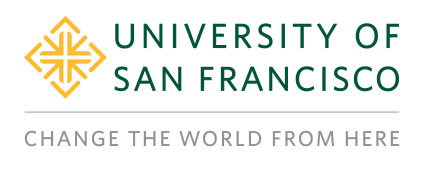
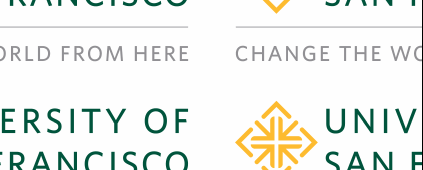
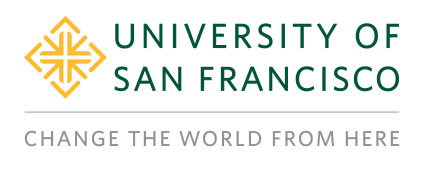
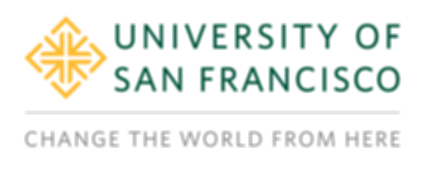
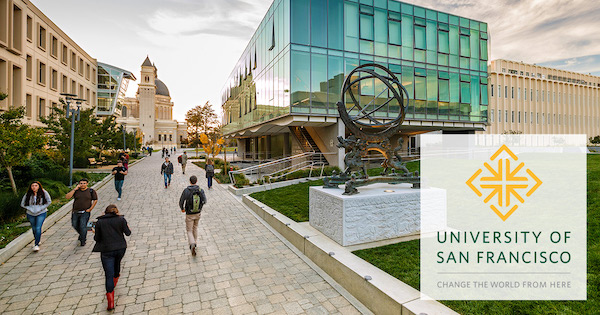
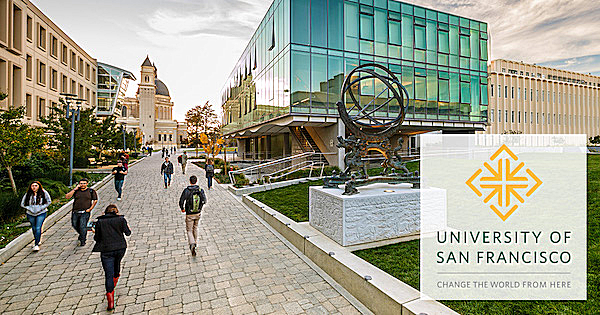
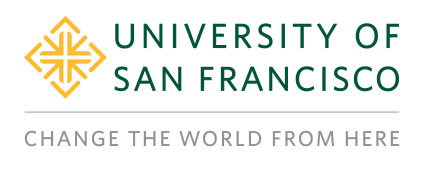
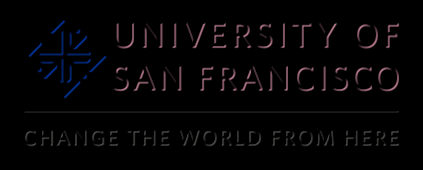
Extra credit options - due 4/2/19:
def scaleLarger(int factor) - scales the image by making a larger image as specified by the parameter factor. If factor is 2, for example, the image will be doubled in size. The new image will be saved to a file scaled.png. (3 points)
Submitting the project
Please turn in the following for the project.
- imageoperations.py
- README file